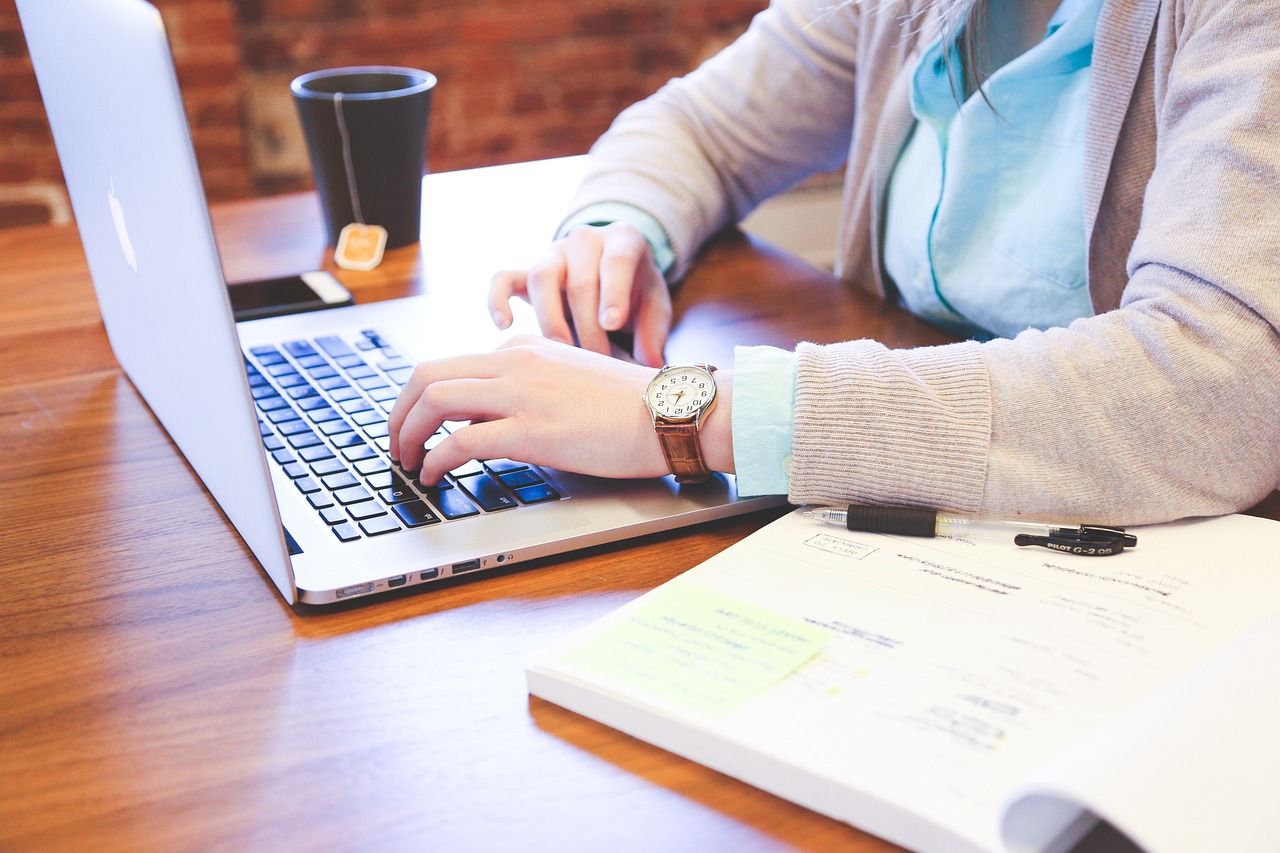
Python stands out as a powerful and versatile programming language renowned for its simplicity and readability. While mastering the basics of Python is crucial, advancing beyond foundational knowledge opens doors to tackling more complex projects and enhancing career opportunities. This tutorial delves into advanced Python programming concepts, focusing on essential topics that go beyond the basics. By the end of this guide, you’ll gain a deeper understanding of Python’s capabilities, empowering you to write more efficient and sophisticated code.
Python Data Types and Variables
Python’s versatility is enhanced by its robust Python data types and efficient variables in Python management. Mastering these foundational elements is crucial to unlocking Python’s full potential in your projects.
1.1 Understanding Python Data Types
Python supports a variety of data types that cater to different programming needs:
-
Integer (int): Used for whole numbers without decimals.
-
Float (float): Represents numbers with decimal points.
-
String (str): Handles textual data enclosed within quotes.
-
List: A versatile collection that can contain different types of elements.
-
Tuple: Similar to lists but immutable once created.
-
Dictionary (dict): Stores data in key-value pairs, offering fast lookup times.
-
Set: Unordered collections of unique elements, useful for mathematical operations.
Each data type serves specific purposes, whether you’re manipulating data structures, performing calculations, or managing complex datasets.
Examples and Use Cases
Integers and Floats: Ideal for numerical operations, such as arithmetic calculations or data analytics where precision matters.
python
Copy code
# Example of integer and float usage
age = 25
average_score = 89.5
-
Strings: Essential for handling textual data and formatting output.
# Example of string usage
name = “Alice”
greeting = f”Hello, {name}!”
-
Lists and Tuples: Valuable for storing sequences of data, with lists offering flexibility and tuples ensuring data integrity.
# Example of list and tuple usage
colors = [“red”, “green”, “blue”]
dimensions = (1920, 1080)
-
Dictionaries: Efficient for mapping keys to values, facilitating quick data retrieval.
# Example of dictionary usage
student = {
“name”: “Bob”,
“age”: 23,
“major”: “Computer Science”
}
Sets: Useful for operations requiring unique elements or mathematical computations.
# Example of set usage
unique_numbers = {1, 2, 3, 4, 5}
Understanding when and how to use each data type optimally empowers developers to write cleaner, more efficient code tailored to specific tasks and requirements. Mastery of Python’s data types is fundamental for progressing to more advanced programming techniques covered in subsequent sections.
Section 2: Functional Programming in Python
Python’s support for functional programming paradigms empowers developers to write concise, modular, and reusable code by focusing on functions as first-class citizens.
2.1 Lambda Functions and Functional Programming Concepts
Lambda functions, also known as anonymous functions, are a cornerstone of functional programming in Python. They allow for the creation of small, one-line functions without needing to define them using the def keyword.
Explanation of Lambda Functions
Lambda functions are defined using the lambda keyword, followed by parameters and an expression. They are particularly useful in situations where a small function is needed temporarily or where functions are passed as arguments to higher-order functions.
Example of Lambda Functions
# Example of a lambda function
square = lambda x: x ** 2
result = square(5) # Returns 25
Functional Programming Concepts
Functional programming encourages immutable data and avoids side effects, emphasizing the use of higher-order functions like map, filter, and reduce.
Examples of Higher-Order Functions
map Function: Applies a function to all items in an iterable.
# Example of map function
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x ** 2, numbers)) # Returns [1, 4, 9, 16, 25]
-
filter Function: Filters elements based on a function’s condition.
# Example of filter function
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) # Returns [2, 4]
-
reduce Function: Performs a cumulative operation on a list.
from functools import reduce
# Example of reduce function
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers) # Returns 120 (1 * 2 * 3 * 4 * 5)
Understanding and effectively using these functional programming concepts not only simplifies code but also enhances its readability and maintainability, making it a powerful tool in Python development.
Section 3: Object-Oriented Programming (OOP) in Python
Python’s object-oriented programming capabilities allow developers to structure code into reusable and understandable components using classes and objects.
3.1 Inheritance and Polymorphism
Inheritance enables the creation of new classes that inherit attributes and methods from existing ones, promoting code reuse and organization.
Overview of Inheritance
-
Single Inheritance: A class inherits from a single parent class.
-
Multiple Inheritance: A class inherits from multiple parent classes.
-
Multilevel Inheritance: Classes are derived from other derived classes.
Example of Inheritance
# Example of inheritance
class Animal:
def speak(self):
print(“Animal speaks”)
class Dog(Animal):
def bark(self):
print(“Dog barks”)
# Creating an instance of Dog
dog = Dog()
dog.speak() # Outputs: “Animal speaks”
dog.bark() # Outputs: “Dog barks”
Polymorphism in Python
Polymorphism allows methods to be overridden in subclasses, providing different implementations while retaining the same method signature.
Examples of Polymorphism
# Example of polymorphism
class Bird:
def fly(self):
print(“Bird flies”)
class Sparrow(Bird):
def fly(self):
print(“Sparrow flies”)
# Creating an instance of Sparrow
sparrow = Sparrow()
sparrow.fly() # Outputs: “Sparrow flies”
Understanding inheritance and polymorphism enables developers to create more flexible and scalable codebases, enhancing code reusability and maintainability.
3.2 Advanced Class Features
Python’s class system offers additional features such as class methods, static methods, and instance methods, each serving distinct purposes in object-oriented programming.
Class Methods, Static Methods, and Instance Methods
Class Methods: Associated with the class rather than instances. They can access and modify class state.
# Example of class method
class MyClass:
count = 0
@classmethod
def increase_count(cls):
cls.count += 1
@classmethod
def get_count(cls):
return cls.count
MyClass.increase_count()
print(MyClass.get_count()) # Outputs: 1
-
Static Methods: Independent of class and instance state, often used for utility functions.
# Example of static method
class MathUtils:
@staticmethod
def add(x, y):
return x + y
result = MathUtils.add(3, 5) # Returns 8
-
Instance Methods: Operate on instances of a class and can access instance variables.
# Example of instance method
class Car:
def __init__(self, brand):
self.brand = brand
def display_brand(self):
print(f”Brand: {self.brand}”)
my_car = Car(“Toyota”)
my_car.display_brand() # Outputs: “Brand: Toyota”
-
Mastering these advanced class features empowers developers to design sophisticated object-oriented solutions, leveraging Python’s rich capabilities for complex application development.