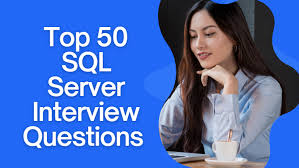
1. Introduction
Understanding data structures in Python is essential for anyone preparing for technical interviews, especially for freshers aiming to secure their first job in the tech industry. This guide is designed to help you master key data structures and prepare you for Python interview questions for freshers. By the end of this guide, you’ll be equipped with the knowledge and confidence to tackle any data structure-related question in your interviews.
2. Basic Data Structures in Python
Lists
Lists are one of the most versatile data structures in Python. They allow you to store an ordered collection of items, which can be of any type. Lists are mutable, meaning you can change their content without changing their identity.
Basic Operations:
- Creating a list: my_list = [1, 2, 3, 4, 5]
- Accessing elements: my_list[0] (returns 1)
- Adding elements: my_list.append(6)
- Removing elements: my_list.remove(3)
Use Cases:
- Storing a collection of related items
- Iterating over a sequence of elements
Tuples
Tuples are similar to lists but are immutable, meaning once created, their content cannot be changed. This makes them useful for fixed collections of items.
Basic Operations:
- Creating a tuple: my_tuple = (1, 2, 3)
- Accessing elements: my_tuple[1] (returns 2)
Use Cases:
- Storing a sequence of items that should not change
- Returning multiple values from a function
Sets
Sets are unordered collections of unique items. They are useful for membership testing and eliminating duplicate entries.
Basic Operations:
- Creating a set: my_set = {1, 2, 3}
- Adding elements: my_set.add(4)
- Removing elements: my_set.remove(2)
Use Cases:
- Removing duplicates from a list
- Checking for membership
Dictionaries
Dictionaries are collections of key-value pairs. They are useful for storing data that can be quickly retrieved using a unique key.
Basic Operations:
- Creating a dictionary: my_dict = {‘name’: ‘Alice’, ‘age’: 25}
- Accessing values: my_dict[‘name’] (returns ‘Alice’)
- Adding key-value pairs: my_dict[‘city’] = ‘New York’
- Removing key-value pairs: del my_dict[‘age’]
Use Cases:
- Storing and retrieving data by key
- Representing structured data
3. Advanced Data Structures
Stacks
A stack follows the Last In, First Out (LIFO) principle. You can implement a stack using a list in Python.
Implementation:
python
Copy code
stack = []
stack.append(‘a’)
stack.append(‘b’)
stack.append(‘c’)
stack.pop() # returns ‘c’
Use Cases:
- Undo mechanisms in text editors
- Backtracking algorithms
Queues
A queue follows the First In, First Out (FIFO) principle. You can implement a queue using a list or collections.deque.
Implementation:
python
Copy code
from collections import deque
queue = deque()
queue.append(‘a’)
queue.append(‘b’)
queue.popleft() # returns ‘a’
Use Cases:
- Task scheduling
- Breadth-first search in graphs
Linked Lists
A linked list is a sequence of nodes where each node points to the next node in the sequence. They come in two varieties: singly and doubly linked lists.
Implementation:
python
Copy code
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
Use Cases:
- Dynamic memory allocation
- Implementing other data structures like stacks and queues
Trees
Trees are hierarchical data structures with a root node and child nodes forming a parent-child relationship. Binary trees, where each node has at most two children, are particularly common.
Implementation:
python
Copy code
class TreeNode:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
Use Cases:
- Representing hierarchical data
- Binary search trees for efficient searching and sorting
4. Algorithms and Data Structures
Sorting Algorithms
Sorting algorithms are essential for organizing data. Common algorithms include quick sort, merge sort, and bubble sort.
Quick Sort:
python
Copy code
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
Time Complexities:
- Quick sort: O(n log n) on average
- Merge sort: O(n log n)
- Bubble sort: O(n^2)
Searching Algorithms
Searching algorithms help you find elements within data structures. Common algorithms include binary search and linear search.
Binary Search:
python
Copy code
def binary_search(arr, x):
low = 0
high = len(arr) – 1
mid = 0
while low <= high:
mid = (high + low) // 2
if arr[mid] < x:
low = mid + 1
elif arr[mid] > x:
high = mid – 1
else:
return mid
return -1
Time Complexities:
- Binary search: O(log n)
- Linear search: O(n)
5. Practical Interview Tips
To succeed in Python interviews, particularly those focusing on data structures, follow these practical tips:
- Analyze the problem statement: Understand what is being asked before jumping into coding.
- Choose the right data structure: Select a data structure that best fits the problem requirements.
- Write clean and efficient code: Aim for readability and optimize for performance.
- Avoid common mistakes: Be mindful of edge cases and off-by-one errors.
6. Practice Problems
To reinforce your understanding, practice with the following sample interview questions and problems:
- Reverse a linked list.
- Find the largest element in a binary search tree.
- Implement a queue using two stacks.
- Sort an array using quick sort.
For each problem, start with a plan, write the code, and then test thoroughly. Use platforms like LeetCode, HackerRank, and CodeSignal for additional practice.
7. Conclusion
Mastering data structures in Python is a crucial step toward acing Python interview questions for freshers. Regular practice and a clear understanding of each data structure’s strengths and weaknesses will significantly boost your confidence and performance in interviews.
8. Additional Resources
To further enhance your skills, consider the following resources:
- Books:
- “Data Structures and Algorithms in Python” by Michael T. Goodrich, Roberto Tamassia, and Michael H. Goldwasser
- “Python Data Structures and Algorithms” by Benjamin Baka
- Online Courses:
- Coursera: Data Structures and Algorithms Specialization
- Udacity: Data Structures and Algorithms Nanodegree
- Coding Platforms:
- LeetCode
- HackerRank
- CodeSignal
By leveraging these resources and consistently practicing, you’ll be well on your way to mastering data structures in Python and excelling in your technical interviews.